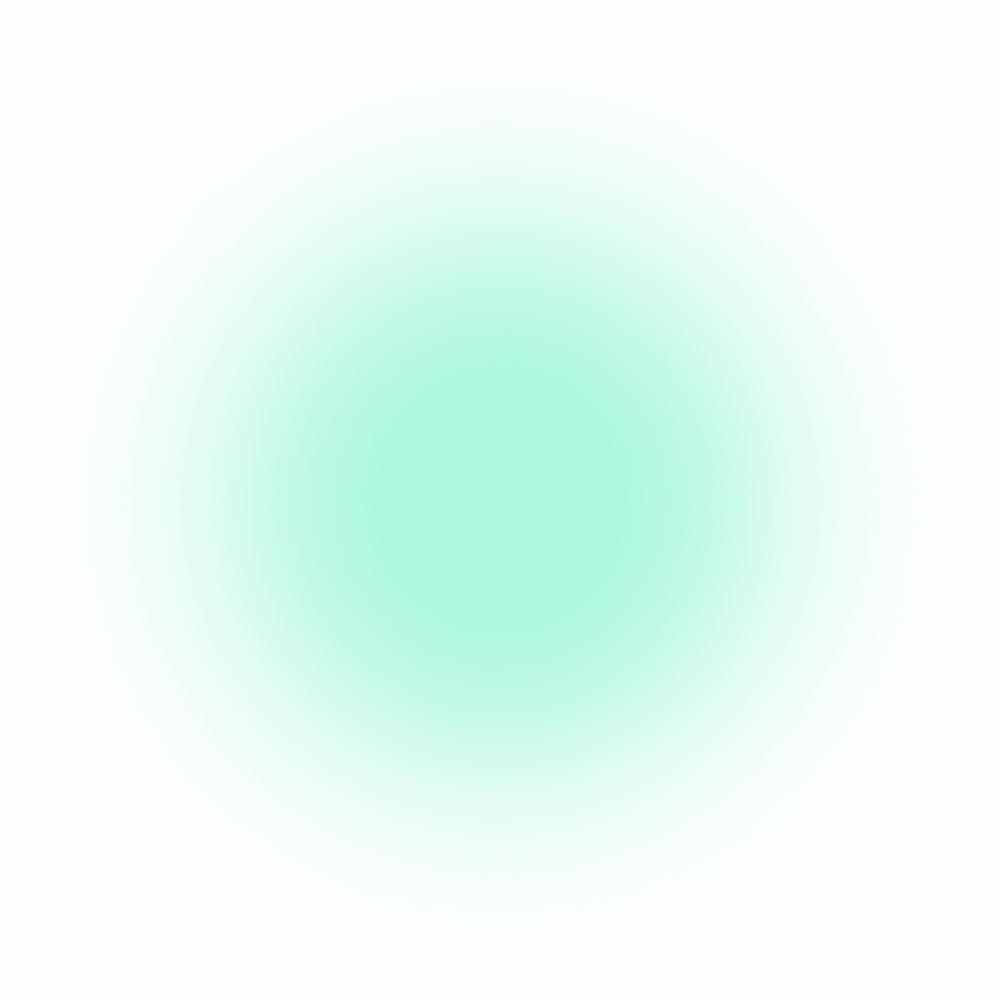
API
-
HTTP MethodPOST
-
API URLhttps://greenlike.pro/api/v2
-
API KeyGet an API key on the Account page
-
Response formatJSON
Service list
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionservices
Example response
[ { "service": 1, "name": "Followers", "type": "Default", "category": "First Category", "rate": "0.90", "min": "50", "max": "10000", "refill": true, "cancel": true }, { "service": 2, "name": "Comments", "type": "Custom Comments", "category": "Second Category", "rate": "8", "min": "10", "max": "1500", "refill": false, "cancel": true } ]
Add order
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionadd
-
Parametersservice
-
DescriptionService ID
-
Parameterslink
-
DescriptionLink to page
-
Parametersquantity
-
DescriptionNeeded quantity
-
Parametersruns (optional)
-
DescriptionRuns to deliver
-
Parametersinterval (optional)
-
DescriptionInterval in minutes
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionadd
-
Parametersservice
-
DescriptionService ID
-
Parameterslink
-
DescriptionLink to page
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionadd
-
Parametersservice
-
DescriptionService ID
-
Parameterslink
-
DescriptionLink to page
-
Parametersquantity
-
DescriptionNeeded quantity
-
Parameterskeywords
-
DescriptionKeywords list separated by \r\n or \n
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionadd
-
Parametersservice
-
DescriptionService ID
-
Parameterslink
-
DescriptionLink to page
-
Parameterscomments
-
DescriptionComments list separated by \r\n or \n
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionadd
-
Parametersservice
-
DescriptionService ID
-
Parameterslink
-
DescriptionLink to page
-
Parametersquantity
-
DescriptionNeeded quantity
-
Parametersusername
-
DescriptionURL to scrape followers from
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionadd
-
Parametersservice
-
DescriptionService ID
-
Parametersusername
-
DescriptionUsername
-
Parametersmin
-
DescriptionQuantity min
-
Parametersmax
-
DescriptionQuantity max
-
Parametersposts (optional)
-
DescriptionUse this parameter if you want to limit the number of new (future) posts that will be parsed and for which orders will be created. If posts parameter is not set, the subscription will be created for an unlimited number of posts.
-
Parametersold_posts (optional)
-
DescriptionNumber of existing posts that will be parsed and for which orders will be created, can be used if this option is available for the service.
-
Parametersdelay
-
DescriptionDelay in minutes. Possible values: 0, 5, 10, 15, 20, 30, 40, 50, 60, 90, 120, 150, 180, 210, 240, 270, 300, 360, 420, 480, 540, 600
-
Parametersexpiry (optional)
-
DescriptionExpiry date. Format d/m/Y
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionadd
-
Parametersservice
-
DescriptionService ID
-
Parameterslink
-
DescriptionLink to page
-
Parametersquantity
-
DescriptionNeeded quantity
-
Parametersruns (optional)
-
DescriptionRuns to deliver
-
Parametersinterval (optional)
-
DescriptionInterval in minutes
-
Parameterscountry
-
DescriptionCountry code or full country name. Format: "US" or "United States"
-
Parametersdevice
-
DescriptionDevice name. 1 - Desktop, 2 - Mobile (Android), 3 - Mobile (IOS), 4 - Mixed (Mobile), 5 - Mixed (Mobile & Desktop)
-
Parameterstype_of_traffic
-
Description1 - Google Keyword, 2 - Custom Referrer, 3 - Blank Referrer
-
Parametersgoogle_keyword
-
Descriptionrequired if type_of_traffic = 1
-
Parametersreferring_url
-
Descriptionrequired if type_of_traffic = 2
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionadd
-
Parametersservice
-
DescriptionService ID
-
Parameterslink
-
DescriptionLink to page
-
Parameterscomments
-
DescriptionComments list separated by \r\n or \n
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionadd
-
Parametersservice
-
DescriptionService ID
-
Parameterslink
-
DescriptionLink to page
-
Parametersquantity
-
DescriptionNeeded quantity
-
Parametersanswer_number
-
DescriptionAnswer number of the poll
Example response
{ "order": 23501 }
Order status
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionstatus
-
Parametersorder
-
DescriptionOrder ID
Example response
{ "charge": "0.27819", "start_count": "3572", "status": "Partial", "remains": "157", "currency": "USD" }
Multiple orders status
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionstatus
-
Parametersorders
-
DescriptionOrder IDs (separated by a comma, up to 100 IDs)
Example response
{ "1": { "charge": "0.27819", "start_count": "3572", "status": "Partial", "remains": "157", "currency": "USD" }, "10": { "error": "Incorrect order ID" }, "100": { "charge": "1.44219", "start_count": "234", "status": "In progress", "remains": "10", "currency": "USD" } }
Create refill
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionrefill
-
Parametersorder
-
DescriptionOrder ID
Example response
{ "refill": "1" }
Create multiple refill
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionrefill
-
Parametersorders
-
DescriptionOrder IDs (separated by a comma, up to 100 IDs)
Example response
[ { "order": 1, "refill": 1 }, { "order": 2, "refill": 2 }, { "order": 3, "refill": { "error": "Incorrect order ID" } } ]
Get refill status
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionrefill_status
-
Parametersrefill
-
DescriptionRefill ID
Example response
{ "status": "Completed" }
Get multiple refill status
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionrefill_status
-
Parametersrefills
-
DescriptionRefill IDs (separated by a comma, up to 100 IDs)
Example response
[ { "refill": 1, "status": "Completed" }, { "refill": 2, "status": "Rejected" }, { "refill": 3, "status": { "error": "Refill not found" } } ]
Create cancel
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptioncancel
-
Parametersorders
-
DescriptionOrder IDs (separated by a comma, up to 100 IDs)
Example response
[ { "order": 9, "cancel": { "error": "Incorrect order ID" } }, { "order": 2, "cancel": 1 } ]
User balance
-
Parameterskey
-
DescriptionYour API key
-
Parametersaction
-
Descriptionbalance
Example response
{ "balance": "100.84292", "currency": "USD" }